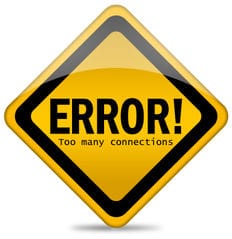
Solving Google Cloud's "Too Many Connections" Problem: A Function-to-Function Call Solution
Published – Jan 1, 2025
Read Length – 1 minute
The Challenge with Google Cloud Connections
Understanding the Root Cause
Google Cloud runs each endpoint in a separate container, which initially seems great for scalability. However, here’s the catch: each container maintains its own database connections. With 500 endpoints, you could suddenly find yourself managing thousands of open MySQL connections – hardly an optimal scenario.
The traditional solutions present their own problems:
- Closing and reopening connections hurts performance
- Limiting endpoint initialization reduces flexibility
- Managing connection pools adds complexity
A Novel Solution: HTTPSCallable Functions
Rather than wrestling with connection management across multiple endpoints, what if we could centralize database operations through a single, callable function? Enter HTTPSCallable functions – a powerful but underutilized feature of Google Cloud.
How It Works
Instead of each function maintaining its own database connection, we create a single HTTPSCallable function that handles all database operations. Other functions call this centralized endpoint, dramatically reducing the number of active database connections while maintaining high performance.
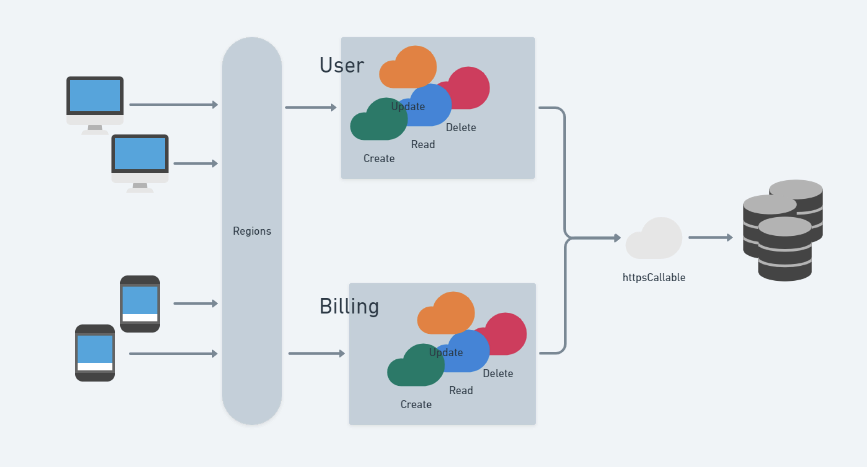
Implementation Guide
1. Creating the Database Handler
Javascript
// index.js
let _dbmodule;
exports.crud = functions.https.onCall(async (data, context) => {
if(!_dbmodule) {
_dbmodule = require("./db");
}
const [op,...rest] = data;
try {
return await _dbmodule[op](...rest)
} catch (e) {
throw e
}
});
2. Setting Up the Database Interface
Javascript
// db.js
export default {
create: async (data) => await db.create(data),
read: async (id) => await db.query({id}),
update: async (id,data) => await db.update({id,$set:data}),
delete: async(id) => await db.update({id,$set:{deleted:1}})
}
3. Creating the HTTPSCallable Module
Javascript
// httpsCallable.js
import axios from 'axios';
module.exports = function(name,context) {
const {protocol,headers} = context.rawRequest;
const host = headers['x-forwardedfor-host'] || headers.host;
const url = `${protocol}://${host}/${name}`;
return async (...rest) => {
const config = {
method: 'post',
url,
data: JSON.stringify({data:rest}),
headers: {
'Content-Type': 'application/json',
'Authorization': headers.authorization,
'Connection': 'keep-alive',
'Cache-control': 'no-cache'
}
};
try {
const {data:{result}} = await axios(config);
return result;
} catch(e) {
throw e;
}
}
}
Benefits and Considerations
Advantages
- Centralized connection management
- Reduced database connection overhead
- Improved scalability
- Better security control
- Simplified maintenance
Potential Drawbacks
- Slight cold-start delay
- Additional layer of abstraction
- Learning curve for new team members
Best Practices
- Cache your module imports to reduce cold-start times
- Implement proper error handling
- Consider adding a feature flag for direct/remote calls
- Monitor performance metrics
- Maintain clear documentation
When to Use This Pattern
This approach is particularly valuable when:
- You need to manage remote connections efficiently
- Your database has connection limits
- Security requirements demand centralized database access
- You’re optimizing resource utilization
Conclusion
While function-to-function calls might seem counterintuitive at first, they offer a elegant solution to the database connection challenge in Google Cloud. By centralizing database operations through a single HTTPSCallable function, you can maintain high performance while keeping connection counts manageable.
Remember, sometimes the best solutions aren’t the most obvious ones. This pattern has proven effective in production environments, particularly for applications using cost-efficient database resources with connection limits.
Let us help!
Related Services: Explore our project management services to help streamline your projects and prevent budget explosions.